pytorch中LN(LayerNorm)及Relu和其变相的输出操作
主要就是了解一下pytorch中的使用layernorm这种归一化之后的数据变化,以及数据使用relu,prelu,leakyrelu之后的变化。
import torch
import torch.nn as nn
import torch.nn.functional as F
class model(nn.Module):
def __init__(self):
super(model, self).__init__()
self.LN=nn.LayerNorm(10,eps=0,elementwise_affine=True)
self.PRelu=nn.PReLU(init=0.25)
self.Relu=nn.ReLU()
self.LeakyReLU=nn.LeakyReLU(negative_slope=0.01,inplace=False)
def forward(self,input ):
out=self.LN(input)
print("LN:",out)
out1=self.PRelu(out)
print("PRelu:",out1)
out2=self.Relu(out)
print("Relu:",out2)
out3=self.LeakyReLU(out)
print("LeakyRelu:",out3)
return out
tensor=torch.tensor([-0.9,0.1,0,-0.1,0.9,-0.4,0.9,-0.5,0.8,0.1])
net=model()
print(tensor)
net(tensor)
输出:
tensor([-0.9000, 0.1000, 0.0000, -0.1000, 0.9000, -0.4000, 0.9000, -0.5000, 0.8000, 0.1000]) LN: tensor([-1.6906, 0.0171, -0.1537, -0.3245, 1.3833, -0.8368, 1.3833, -1.0076, 1.2125, 0.0171], grad_fn=<NativeLayerNormBackward>) Relu: tensor([0.0000, 0.0171, 0.0000, 0.0000, 1.3833, 0.0000, 1.3833, 0.0000, 1.2125, 0.0171], grad_fn=<ReluBackward0>) PRelu: tensor([-0.4227, 0.0171, -0.0384, -0.0811, 1.3833, -0.2092, 1.3833, -0.2519, 1.2125, 0.0171], grad_fn=<PreluBackward>) LeakyRelu: tensor([-0.0169, 0.0171, -0.0015, -0.0032, 1.3833, -0.0084, 1.3833, -0.0101, 1.2125, 0.0171], grad_fn=<LeakyReluBackward0>)
从上面可以看出,这个LayerNorm的归一化,并不是将数据限定在0-1之间,也没有进行一个类似于高斯分布一样的分数,只是将其进行了一个处理,对应的数值得到了一些变化,相同数值的变化也是相同的。
Relu的则是单纯将小于0的数变成了0,减少了梯度消失的可能性
PRelu是一定程度上的保留了负值,根据init给的值。
LeakyRelu也是一定程度上保留负值,不过比较小,应该是根据negative_slope给的值。
补充:PyTorch学习之归一化层(BatchNorm、LayerNorm、InstanceNorm、GroupNorm)
BN,LN,IN,GN从学术化上解释差异:
BatchNorm:batch方向做归一化,算NHW的均值,对小batchsize效果不好;BN主要缺点是对batchsize的大小比较敏感,由于每次计算均值和方差是在一个batch上,所以如果batchsize太小,则计算的均值、方差不足以代表整个数据分布
LayerNorm:channel方向做归一化,算CHW的均值,主要对RNN作用明显;
InstanceNorm:一个channel内做归一化,算H*W的均值,用在风格化迁移;因为在图像风格化中,生成结果主要依赖于某个图像实例,所以对整个batch归一化不适合图像风格化中,因而对HW做归一化。可以加速模型收敛,并且保持每个图像实例之间的独立。
GroupNorm:将channel方向分group,然后每个group内做归一化,算(C//G)HW的均值;这样与batchsize无关,不受其约束。
SwitchableNorm是将BN、LN、IN结合,赋予权重,让网络自己去学习归一化层应该使用什么方法。
1 BatchNorm
torch.nn.BatchNorm1d(num_features, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
torch.nn.BatchNorm2d(num_features, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
torch.nn.BatchNorm3d(num_features, eps=1e-05, momentum=0.1, affine=True, track_running_stats=True)
参数:
num_features: 来自期望输入的特征数,该期望输入的大小为'batch_size x num_features [x width]'
eps: 为保证数值稳定性(分母不能趋近或取0),给分母加上的值。默认为1e-5。
momentum: 动态均值和动态方差所使用的动量。默认为0.1。
affine: 布尔值,当设为true,给该层添加可学习的仿射变换参数。
track_running_stats:布尔值,当设为true,记录训练过程中的均值和方差;
实现公式:
track_running_stats:布尔值,当设为true,记录训练过程中的均值和方差;
实现公式:
2 GroupNorm
torch.nn.GroupNorm(num_groups, num_channels, eps=1e-05, affine=True)
参数:
num_groups:需要划分为的groups
num_features:来自期望输入的特征数,该期望输入的大小为'batch_size x num_features [x width]'
eps:为保证数值稳定性(分母不能趋近或取0),给分母加上的值。默认为1e-5。
momentum:动态均值和动态方差所使用的动量。默认为0.1。
affine:布尔值,当设为true,给该层添加可学习的仿射变换参数。
实现公式:
3 InstanceNorm
torch.nn.InstanceNorm1d(num_features, eps=1e-05, momentum=0.1, affine=False, track_running_stats=False)
torch.nn.InstanceNorm2d(num_features, eps=1e-05, momentum=0.1, affine=False, track_running_stats=False)
torch.nn.InstanceNorm3d(num_features, eps=1e-05, momentum=0.1, affine=False, track_running_stats=False)
参数:
num_features:来自期望输入的特征数,该期望输入的大小为'batch_size x num_features [x width]'
eps:为保证数值稳定性(分母不能趋近或取0),给分母加上的值。默认为1e-5。
momentum:动态均值和动态方差所使用的动量。默认为0.1。
affine:布尔值,当设为true,给该层添加可学习的仿射变换参数。
track_running_stats:布尔值,当设为true,记录训练过程中的均值和方差;
实现公式:
4 LayerNorm
torch.nn.LayerNorm(normalized_shape, eps=1e-05, elementwise_affine=True)
参数:
normalized_shape: 输入尺寸
[∗×normalized_shape[0]×normalized_shape[1]×…×normalized_shape[−1]]
eps: 为保证数值稳定性(分母不能趋近或取0),给分母加上的值。默认为1e-5。
elementwise_affine: 布尔值,当设为true,给该层添加可学习的仿射变换参数。
实现公式:
5 LocalResponseNorm
torch.nn.LocalResponseNorm(size, alpha=0.0001, beta=0.75, k=1.0)
参数:
size:用于归一化的邻居通道数
alpha:乘积因子,Default: 0.0001
beta :指数,Default: 0.75
k:附加因子,Default: 1
实现公式:
(资源库 www.zyku.net)
原文链接:https://blog.csdn.net/qq_41487299/article/details/106947938
上一篇:pytorch 实现多个Dataloader同时训练
栏 目:Python教程
本文标题:pytorch中LN(LayerNorm)及Relu和其变相的输出操作
本文地址:https://www.zyku.net/python/9634.html
您可能感兴趣的文章
- 02-08PyTorch梯度裁剪避免训练loss nan的操作
- 02-08我对PyTorch dataloader里的shuffle=True的理解
- 02-08浅谈pytorch中为什么要用 zero_grad() 将梯度清零
- 02-08pytorch DataLoader的num_workers参数与设置大小详解
- 02-08pytorch 带batch的tensor类型图像显示操作
- 02-08解决pytorch中的kl divergence计算问题
- 02-08pytorch 实现在测试的时候启用dropout
- 04-09使用pytorch实现线性回归
- 01-13织梦dedecms内页、详情页中调用文章发
- 01-12dedecms 在内容模板里调用栏目内容即{
- 12-12小米平板5如何关闭屏幕
- 09-25华为手表fitnew设置微信消息通知步骤
- 12-27mtc摩托车驾考助手-mtc摩托车驾考助手
- 10-24vivox60地震预警功能在哪里
- 09-17经纬度转详细地址几种方式
- 11-23网易云音乐个人主页背景如何修改
- 07-05Linux cu命令
- 04-15荣耀v40轻奢版主题样式切换教程
- 02-20真我gtneo相机水印设置教程
- 09-20苹果手机微信无法访问相册怎么办
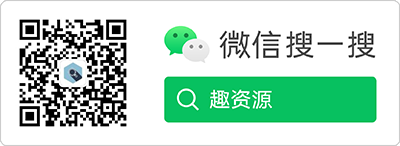
最近更新
阅读排行
猜你喜欢
- 03-28vivoy31s字体样式设置方法
- 12-31人文时刻-人文时刻应用软件功能介绍
- 11-10铁路12306怎么买船票
- 09-19菜鸟裹裹怎么同时删除多个地址
- 01-12卡娃伊手账本-卡娃伊手账本应用软件功
- 01-18九酷福音-九酷福音应用软件功能介绍
- 07-05Linux uuencode命令
- 03-22华为手机输入法剪贴板打开方法
- 11-02华为手环6开启经期提醒方法介绍
- 01-11时雨天气-时雨天气应用软件功能介绍