Yii框架实现的验证码、登录及退出功能示例
本文实例讲述了Yii框架实现的验证码、登录及退出功能。分享给大家供大家参考,具体如下:
捣鼓了一下午,总算走通了,下面贴出代码。
Model
<?php class Auth extends CActiveRecord { public static function model($className = __CLASS__) { return parent::model($className); } public function tableName() { return '{{auth}}'; } }
注:我的用户表是auth,所以模型是Auth.php
<?php class IndexForm extends CFormModel { public $a_account; public $a_password; public $rememberMe; public $verifyCode; public $_identity; public function rules() { return array( array('verifyCode', 'captcha', 'allowEmpty' => !CCaptcha::checkRequirements(), 'message'=>'请输入正确的验证码'), array('a_account', 'required', 'message' => '用户名必填'), array('a_password', 'required', 'message' => '密码必填'), array('a_password', 'authenticate'), array('rememberMe', 'boolean'), ); } public function authenticate($attribute, $params) { if (!$this->hasErrors()) { $this->_identity = new UserIdentity($this->a_account, $this->a_password); if (!$this->_identity->authenticate()) { $this->addError('a_password', '用户名或密码不存在'); } } } public function login() { if ($this->_identity === null) { $this->_identity = new UserIdentity($this->a_account, $this->a_password); $this->_identity->authenticate(); } if ($this->_identity->errorCode === UserIdentity::ERROR_NONE) { $duration = $this->rememberMe ? 60*60*24*7 : 0; Yii::app()->user->login($this->_identity, $duration); return true; } else { return false; } } public function attributeLabels() { return array( 'a_account' => '用户名', 'a_password' => '密码', 'rememberMe' => '记住登录状态', 'verifyCode' => '验证码' ); } }
注:IndexForm也可以写成LoginForm,只是系统内已经有了,我就没有替换它,同时注意看自己用户表的字段,一般是password和username,而我的是a_account和a_password
Controller
<?php class IndexController extends Controller { public function actions() { return array( 'captcha' => array( 'class' => 'CCaptchaAction', 'width'=>100, 'height'=>50 ) ); } public function actionLogin() { if (Yii::app()->user->id) { echo "<div>欢迎" . Yii::app()->user->id . ",<a href='" . SITE_URL . "admin/index/logout'>退出</a></div>"; } else { $model = new IndexForm(); if (isset($_POST['IndexForm'])) { $model->attributes = $_POST['IndexForm']; if ($model->validate() && $model->login()) { echo "<div>欢迎" . Yii::app()->user->id . ",<a href='" . SITE_URL . "admin/index/logout'>退出</a></div>";exit; } } $this->render('login', array('model' => $model)); } } public function actionLogout() { Yii::app()->user->logout(); $this->redirect(SITE_URL . 'admin/index/login'); } }
注:第一个方法是添加验证码的
view
<meta http-equiv="content-type" content="text/html;charset=utf-8"> <?php $form = $this->beginWidget('CActiveForm', array( 'id' => 'login-form', 'enableClientValidation' => true, 'clientOptions' => array( 'validateOnSubmit' => true ) )); ?> <div class="row"> <?php echo $form->labelEx($model,'a_account'); ?> <?php echo $form->textField($model,'a_account'); ?> <?php echo $form->error($model,'a_account'); ?> </div> <div class="row"> <?php echo $form->labelEx($model,'a_password'); ?> <?php echo $form->passwordField($model,'a_password'); ?> <?php echo $form->error($model,'a_password'); ?> </div> <?php if(CCaptcha::checkRequirements()) { ?> <div class="row"> <?php echo $form->labelEx($model, 'verifyCode'); ?> <?php $this->widget('CCaptcha'); ?> <?php echo $form->textField($model, 'verifyCode'); ?> <?php echo $form->error($model, 'verifyCode'); ?> </div> <?php } ?> <div class="row rememberMe"> <?php echo $form->checkBox($model,'rememberMe'); ?> <?php echo $form->label($model,'rememberMe'); ?> <?php echo $form->error($model,'rememberMe'); ?> </div> <div class="row buttons"> <?php echo CHtml::submitButton('Submit'); ?> </div> <?php $this->endWidget(); ?>
同时修改项目下protected/components下的UserIdentity.php
<?php /** * UserIdentity represents the data needed to identity a user. * It contains the authentication method that checks if the provided * data can identity the user. */ class UserIdentity extends CUserIdentity { /** * Authenticates a user. * The example implementation makes sure if the username and password * are both 'demo'. * In practical applications, this should be changed to authenticate * against some persistent user identity storage (e.g. database). * @return boolean whether authentication succeeds. */ public function authenticate() { /* $users=array( // username => password 'demo'=>'demo', 'admin'=>'admin', ); if(!isset($users[$this->username])) $this->errorCode=self::ERROR_USERNAME_INVALID; elseif($users[$this->username]!==$this->password) $this->errorCode=self::ERROR_PASSWORD_INVALID; else $this->errorCode=self::ERROR_NONE; return !$this->errorCode; */ $user_model = Auth::model()->find('a_account=:name',array(':name'=>$this->username)); if($user_model === null){ $this -> errorCode = self::ERROR_USERNAME_INVALID; return false; } else if ($user_model->a_password !== md5($this -> password)){ $this->errorCode=self::ERROR_PASSWORD_INVALID; return false; } else { $this->errorCode=self::ERROR_NONE; return true; } } }
(资源库 www.zyku.net)
您可能感兴趣的文章
- 07-31ShopXO 后台忘记管理员登录密码如何重置密码
- 05-10python对接ihuyi实现短信验证码发送
- 03-31ssh 远程登录指定端口
- 03-25FastAdmin隐藏后台登录入口地址的方法
- 09-22Docker中使用SSH连接登录CentOS容器的方法
- 08-21帝国CMS7.5火车采集器免登录发布模块功能介绍
- 08-04Xshell利用ssh密钥登录Linux的方法
- 08-02Discuz!升级后Ucenter无法登录的解决方法
- 08-02Discuz后台登录不上了 登录后自动退出的处理方法
- 06-25Ucenter后台登录验证码显示CCCC的解决方法
- 01-08vivox70nfc在哪
- 03-07荣耀50pro截长屏方法
- 11-15掌上英雄联盟绑定角色方法介绍
- 01-12dedecms 5.6 分页样式代码修改方法
- 04-01opporeno5k实现分屏设置方法
- 04-26Nginx实战之反向代理WebSocket的配置
- 01-13蓝天云办公-蓝天云办公应用软件功能介
- 01-12Live Portrait Maker-Live Portrait M
- 07-05Linux tree命令
- 01-15CentOS最小安装后(无桌面版)设置联网
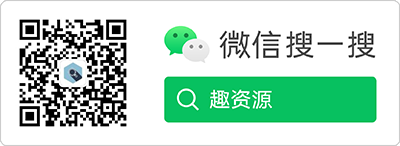
最近更新
阅读排行
猜你喜欢
- 09-09sql server windows nt 64bit 内存占
- 01-12超级清理博士-超级清理博士应用软件功
- 06-012020-01-01T00:00:00.000000Z 日期格
- 01-12屋装宝-屋装宝应用软件功能介绍
- 09-27微信iOS8.0.14更新了什么
- 03-29iPhone给APP单独上锁设置方法
- 08-15华为手机查找手表教程分享
- 01-11内存优化助手-内存优化助手应用软件功
- 04-13oppofindx3设置分屏教程
- 02-06opporeno5振动触感关闭方法